Configuring CORS in Flask
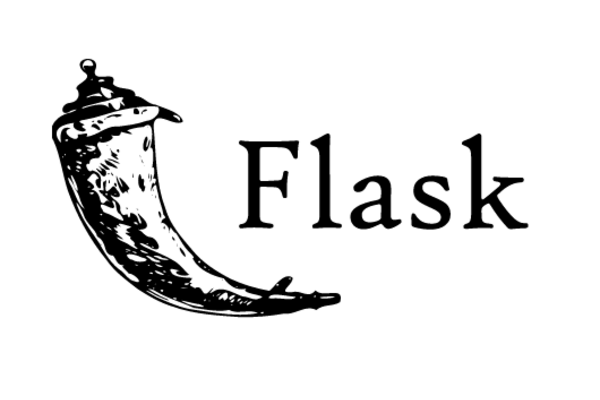
Table of Contents
Introduction
In modern web development, Cross-Origin Resource Sharing (CORS) is a crucial concept, especially when creating APIs with Flask. When your Flask backend and frontend are on different domains, CORS policies come into play to enable secure cross-domain requests. Here’s a guide on setting up CORS in a Flask application.
Understanding CORS in Flask
CORS is a security feature that controls how web applications in one domain interact with resources in another domain. By default, web browsers enforce a same-origin policy for security reasons. However, when your API and client are on different domains, you’ll need to configure CORS to ensure smooth communication.
Installing Flask-CORS
To manage CORS in Flask, we use the Flask-CORS
extension. It’s a simple yet powerful library for handling CORS in Flask applications. Install it using pip:
$ pip install flask-cors
Basic CORS Configuration
Assuming you have a basic Flask app setup, integrating Flask-CORS is straightforward. Import CORS from flask_cors and initialize it with your app:
This configuration enables CORS for all routes on all origins. It’s fine for development, but too open for production.
Customizing CORS Settings
Customizing CORS settings lets you define specific policies for different routes. For example, to restrict CORS to certain origins or specific routes:
Here, only requests to routes
- under /api/
- from https://blockedbycors.dev
- using the GET or POST method
- and with no custom headers except Content-Type are allowed.
This level of customization enhances security by limiting cross-origin requests to trusted sources.
To test your CORS configuration, you can use our CORS Debugger. It’s a free tool that helps you identify and fix CORS issues in your application.
Handling Complex CORS Requests
For more complex scenarios, like non-simple GET or POST requests, or those with custom headers, browsers send a “preflight” request. Flask-CORS handles these out-of-the-box, but you can customize it using additional parameters.
Conclusion
Integrating CORS in your Flask application is essential when your frontend and backend are on different domains. The Flask-CORS extension simplifies this process, providing both basic and advanced configurations to suit your needs. Always tailor your CORS policy to balance functionality and security, especially in a production environment.
With Flask and Flask-CORS, you can confidently build and deploy applications that require secure cross-domain requests, ensuring a seamless experience for your users.
If you'd like to hear about new tools and features we release, sign up for our newsletter. Zero spam.